一、Const 与 Var 的区别
只允许声明事赋值
先定义后使用
不允许重复声明
不支持变量提升
不支持变量提升 console.log(a); //undefined
console.log(b); //Uncaught ReferenceError: b is not defined
var a = 123;而 let / const 会在第一行报
Cannot access 'a' before initialization
,即需要先定义后使用。解决了全局变量污染(不属于顶层对象 window)
暂时性死区 (区块中的 let/const 所声明变量,形成封闭作用域,覆盖作用域之外的同名变量,也就是需要遵循先定义后使用原则)
暂时性死区 var URL = 'https://xxx.com';
if(true) {
URL = 'xxx'; // 此处报错
const xxx;
}此时报:
Uncaught SyntaxError: Missing initializer in const declaration
块级作用域特性
二、Const 变量的可变性
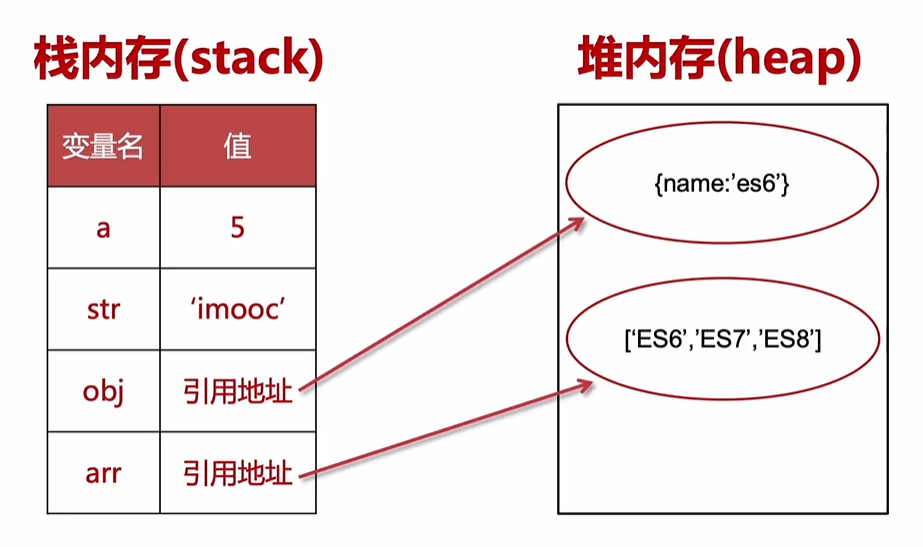
const 定义的引用类型变量,其内容可以更改,可以利用 freeze 冻结保证内容不被改变。
Object.freeze(obj) : 只能冻结一层,例如 const 定义对象中的某个属性为数组,数组中内容依旧可改。
自定义方法遍历实现深层次冻结。
function myFreeze(obj) {
Object.freeze(obj);
Object.keys(obj).forEach(function(key) {
if(typeof obj[key] === 'object')
myFreeze(obj[key]);
});
}
三、箭头函数
- 箭头函数的 this :它会捕获其所在上下文的 this 值, 作为自己的 this 值。
- 参考链接:This 指向详细解析(箭头函数)
箭头函数需要注意的场景:
- 箭头函数作为事件回调函数时。
- 对象中方法的箭头函数时(建议不用)。
- 构造函数中的箭头函数。
- 箭头函数中 arguments 失效。
- 函数原型下的方法。
四、解构赋值
等号左右两边完全匹配
const people = { |
const people = { |
如何正确的使用解构赋值
const sum = ([a, b, c]) => { |
const foo = ({name, age, sex = 'boy'}) => { |
let a = 1; |
const json = '{"name": "xiaoming", "age": 18}'; |
axios.get('./data.json').then(({data: {name, age}}) => { |